Vue Js Convert File Size to MB:Vue js is a popular JavaScript framework for building interactive web applications. One common task when dealing with files in web applications is to convert file sizes from bytes to more human-readable formats like megabytes (MB). In this Vue js example, we will demonstrate how to convert a file size from bytes to megabytes in a user-friendly manner
How to Convert File Size to MB in Vue Js?
To accomplish this task, we’ll create a simple Vue.js example that allows users to select a file and automatically calculates and displays its size in both bytes and megabytes. Here’s the Vue.js code for this functionality:
Vue Js File Size Conversion to MB Example
<div id="app">
<h3>Vue js Convert File Size to MB</h3>
<input type="file" @change="handleFileInputChange" />
<p class="file-size">File Size in Bytes: {{ fileSizeInBytes }} bytes</p>
<p class="file-size">File Size in MB: {{ fileSizeInMB.toFixed(2) }} MB</p>
</div>
In this code, the Vue.js application listens for changes in the file input element. When a file is selected, the handleFileInputChange
method is triggered. This method retrieves the selected file, calculates its size in bytes, and then converts it to megabytes. The file sizes in both bytes and megabytes are stored in Vue.js data properties (fileSizeInBytes
and fileSizeInMB
). The converted file size in megabytes is displayed with two decimal places for better readability
Vue Js File Size Conversion to MB Example
<script>
const app = new Vue({
el: "#app",
data() {
return {
fileSizeInBytes: 0,
fileSizeInMB: 0,
};
},
methods: {
handleFileInputChange(event) {
const file = event.target.files[0];
if (file) {
const fileSizeBytes = file.size;
const fileSizeMB = fileSizeBytes / (1024 * 1024); // Convert to MB
this.fileSizeInBytes = fileSizeBytes;
this.fileSizeInMB = fileSizeMB;
} else {
this.fileSizeInBytes = 0;
this.fileSizeInMB = 0;
}
},
},
})
</script>
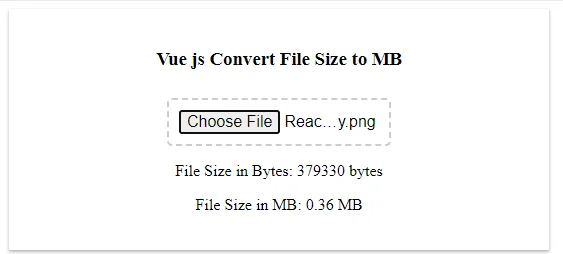
In conclusion, Vue.js makes it straightforward to convert file sizes from bytes to MB in web applications. This example demonstrates how to achieve this functionality, providing a practical solution for dealing with file sizes in a more readable format.